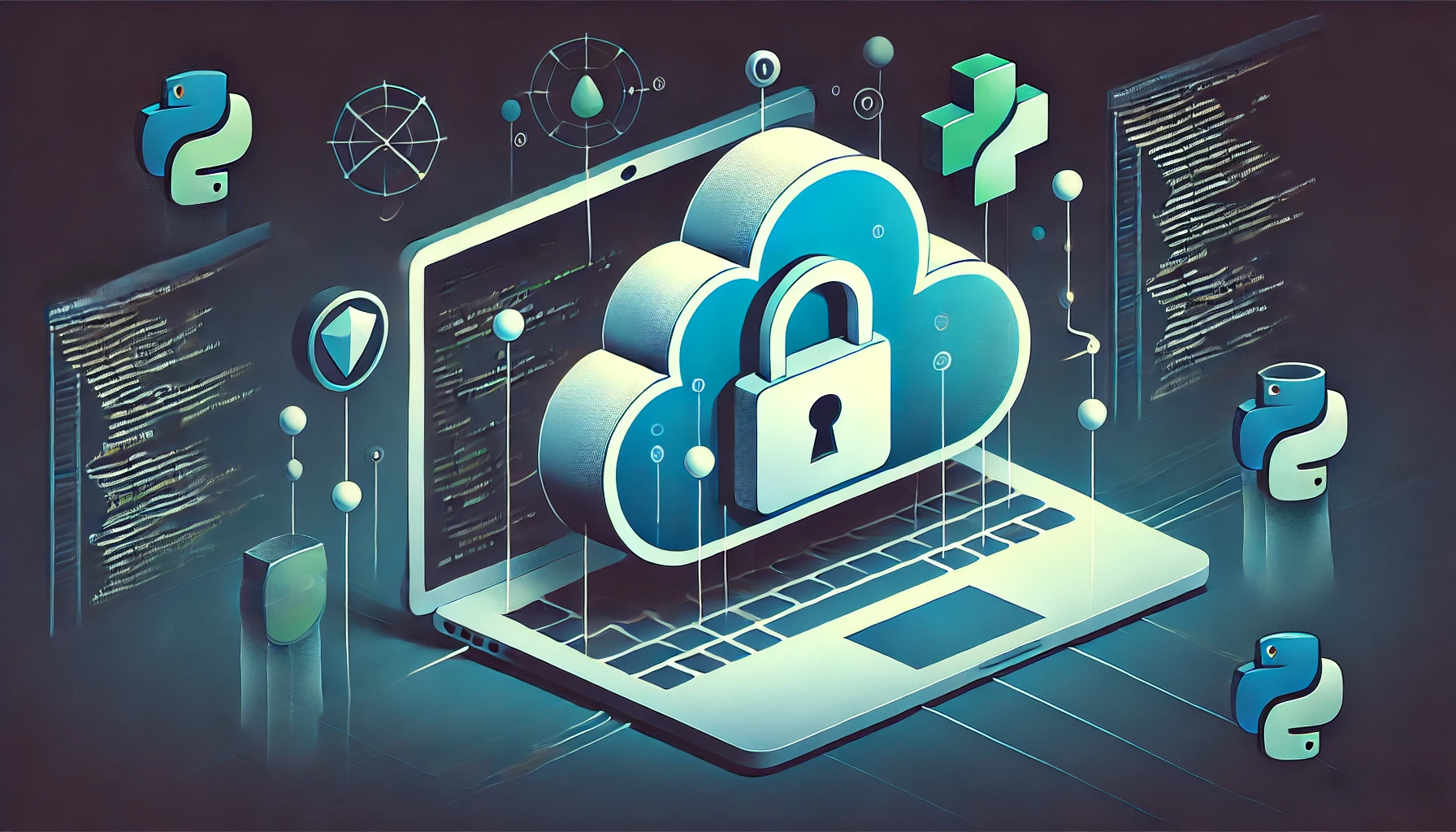
Force Cloudinary Images to Use HTTPS in Django
For developers building Django apps and using Cloudinary for image hosting, you might have encountered console warnings about your images being served over HTTP instead of HTTPS. While modern browsers like Chrome often upgrade HTTP URLs to HTTPS automatically, these warnings can still clutter your DevTools console. As developers, we aim for clean, warning-free consoles—it’s a mark of a well-maintained application.
Beyond aesthetic reasons, serving images over HTTPS is critical for security, ensuring encrypted communication between your app and its users. This guide walks you through three simple ways to configure Cloudinary to always use HTTPS in your Django projects. The right method for you will depend on how your Cloudinary setup is currently configured.
1. If You Are Using the CLOUDINARY_URL Environment Variable
If your project uses the CLOUDINARY_URL
environment variable to configure Cloudinary, the fix is extremely straightforward. You just need to append ?secure=true
to the end of the URL.
Here’s an example:
cloudinary://<API_KEY>:<API_SECRET>@<CLOUD_NAME>?secure=true
By adding ?secure=true
, you ensure that all Cloudinary URLs generated by your app will default to HTTPS.
2. If You Are Using settings.py Configuration
If you’re not using the CLOUDINARY_URL
environment variable and instead have your Cloudinary configuration defined in settings.py, the solution is equally simple. Below is an example of a typical configuration:
# Before
CLOUDINARY_STORAGE = {
'CLOUD_NAME': 'your-cloud-name',
'API_KEY': 'your-api-key',
'API_SECRET': 'your-api-secret',
}
To enable HTTPS, add the 'SECURE': True
key to the CLOUDINARY_STORAGE dictionary:
# After
CLOUDINARY_STORAGE = {
'CLOUD_NAME': 'your-cloud-name',
'API_KEY': 'your-api-key',
'API_SECRET': 'your-api-secret',
'SECURE': True, # Ensures URLs are served over HTTPS
}
This addition forces Cloudinary to serve all image URLs over HTTPS, removing browser warnings and improving security.
3. Force HTTPS Directly in Templates
Another option is to enforce HTTPS directly within your Django templates. This method is particularly useful if you’re generating Cloudinary URLs dynamically in your templates.
Here’s how you can do it:
<img src="{{ image.build_url(secure=True) }}">
The secure=True
parameter ensures that the generated URL is served over HTTPS. This approach provides flexibility if you need to control URL security on a case-by-case basis.
Why Is Enforcing HTTPS Important?
Using HTTPS is more than just a best practice—it’s essential for:
-
Security: Protects user data by encrypting communication.
-
SEO: Search engines prioritize HTTPS websites, which can improve your rankings.
-
User Trust: A secure connection builds trust with your users, indicated by the padlock icon in the browser.
-
Compliance: Many regulations and standards mandate secure communication.
Conclusion
By implementing one of these three methods, you can ensure that Cloudinary images in your Django application are always served over HTTPS. This not only eliminates the console warnings in your DevTools but also enhances the overall security and professionalism of your app.
Choose the method that best aligns with your current setup, and enjoy a cleaner, more secure application environment!