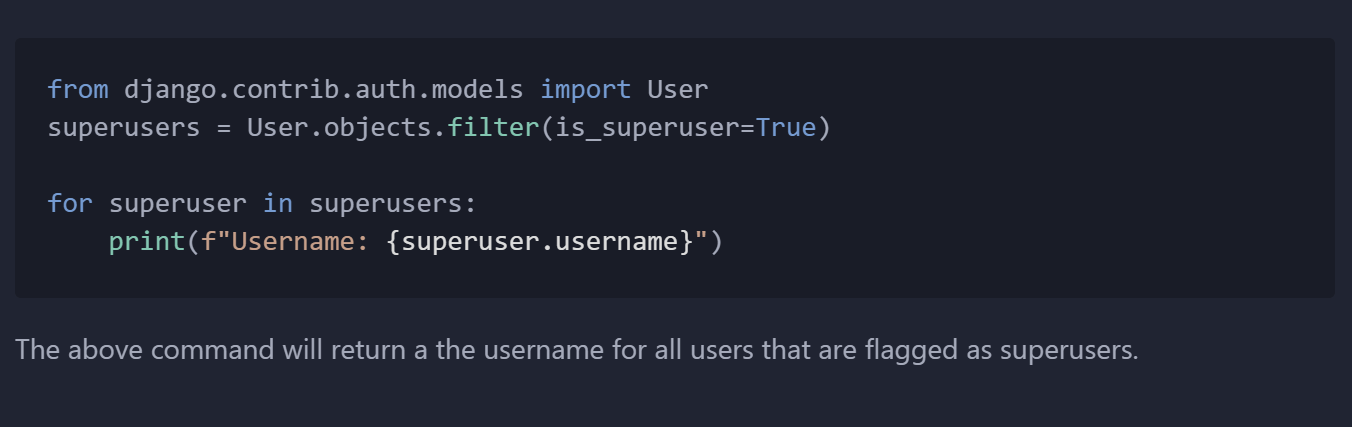
How To: Recover a forgotten superuser username in django
Have you ever opened up your Django project only to realize you can’t remember your superuser username? Don’t worry — you don’t need to create a new superuser. Instead, you can recover the username easily using the Django Shell.
Recovering the Superuser Username
The first step is to navigate into the Django Shell. Run the following command in your terminal:
python manage.py shell
Once inside the shell, execute these lines of code to retrieve all superuser usernames:
from django.contrib.auth.models import User
superusers = User.objects.filter(is_superuser=True)
for superuser in superusers:
print(f"Username: {superuser.username}")
Note: Use Shift + Enter
in the shell to move to the next line without submitting your current line of code.
The above command will return the usernames of all users flagged as superusers in your database.
But What About a Forgotten Superuser Password?
If you’ve forgotten the password for a superuser, it’s even easier to reset. Run this command in your terminal, replacing superuser_username
with the actual username of the account you want to reset:
python manage.py changepassword superuser_username
You’ll be prompted to enter a new password. Once done, your superuser account will be ready to use again.